Table of Contents
Svelte is a modern JavaScript framework designed to make building web applications simpler and more efficient. Unlike traditional frameworks like React or Vue, which use a virtual DOM to manage updates, Svelte shifts much of the work to compile time. Svelte applications are converted into highly optimized vanilla JavaScript at build time, resulting in faster runtime performance and smaller bundle sizes.
Why Choose Svelte for Web Development?
Svelte stands out in the crowded landscape of JavaScript frameworks for several compelling reasons:
- Performance: By compiling components down to efficient, imperative code that directly manipulates the DOM, Svelte eliminates the overhead associated with virtual DOM diffing. This results in faster initial loads and more responsive user interfaces.
- Simplicity: Svelte’s syntax is designed to be intuitive and easy to learn. A minimalistic approach reduces boilerplate code, allowing developers to focus on building features rather than wrestling with the framework itself.
- Developer Experience: Svelte offers a smooth and enjoyable development experience. It provides clear and concise error messages, a powerful interactive tutorial, and a vibrant community. The Svelte DevTools extension helps in debugging and optimizing applications seamlessly.
- Reactive Programming: Svelte’s reactivity model is built into the language itself. This means that state management is more straightforward compared to other frameworks. Reactive statements and variables make it easy to manage and update the state of your application.
- Component-Based Architecture: Like other modern frameworks, Svelte promotes a component-based architecture. This modular approach makes it easier to build, maintain, and scale applications. Components in Svelte are self-contained, reusable, and can be easily composed to create complex user interfaces.
- No Virtual DOM: The absence of a virtual DOM means fewer layers of abstraction between your code and the actual DOM, leading to more predictable and performant applications.
Svelte is a powerful and innovative framework that combines the best aspects of modern web development practices. Its focus on performance, simplicity, and outstanding developer experience makes it an excellent choice for both beginners and experienced developers looking to build efficient and maintainable web applications.
Setting Up Your Development Environment
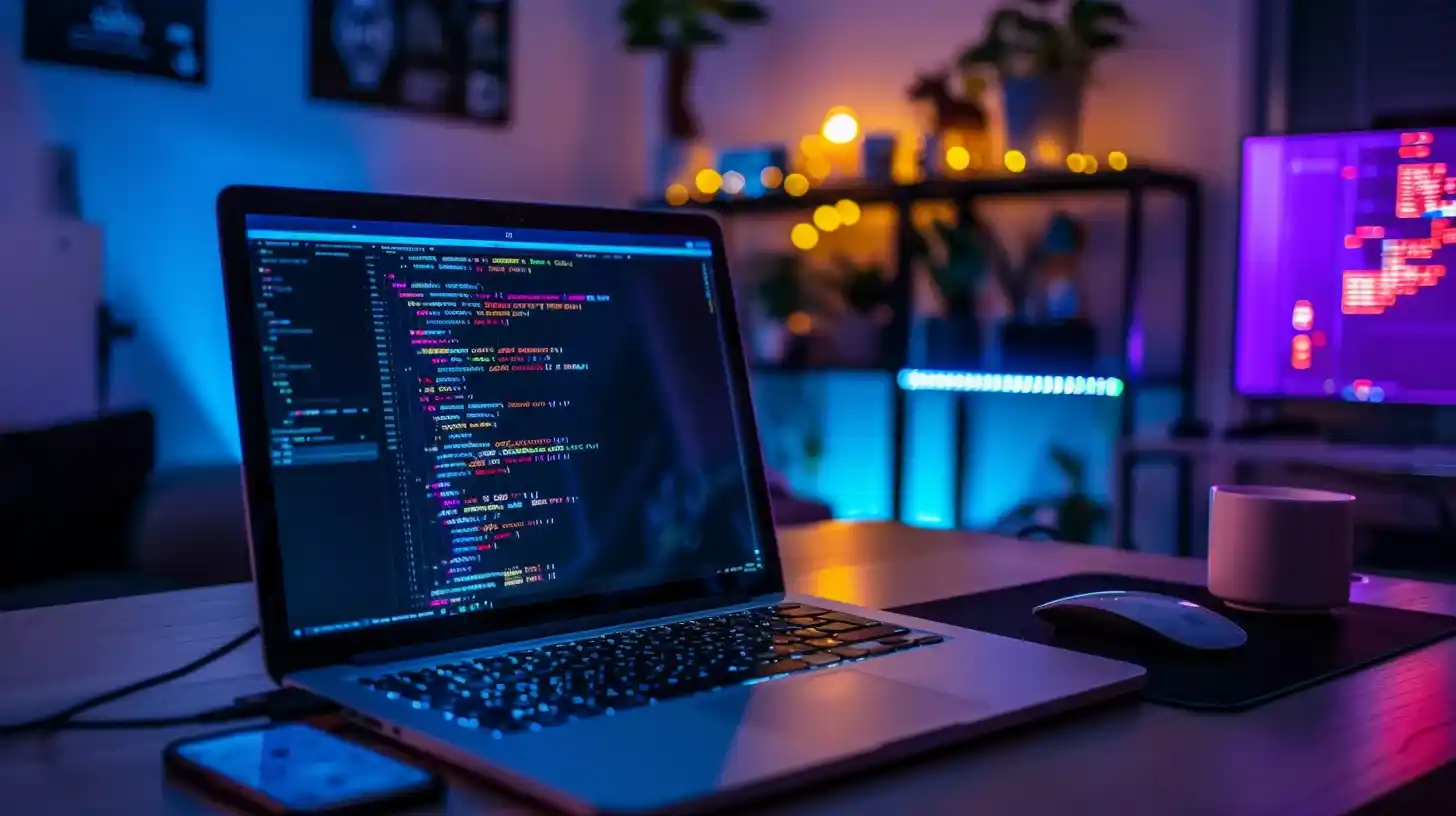
Before diving into Svelte development, it’s essential to set up your development environment properly. This will ensure a smooth and efficient workflow as you build your applications. In this section, we’ll cover the necessary steps to get your environment ready, including installing Node.js and npm (or yarn), setting up a new Svelte project, and configuring your code editor for optimal Svelte development.
Installing Node.js and npm (or yarn)
Svelte relies on Node.js and npm (Node Package Manager) to manage dependencies and run development scripts. Follow these steps to install Node.js and npm on your machine:
- Download Node.js: Visit the official Node.js website and download the latest LTS (Long Term Support) version for your operating system.
- Install Node.js: Run the downloaded installer and follow the on-screen instructions to complete the installation. This process will also install npm automatically.
- Verify Installation: Open your terminal or command prompt and run the following commands to verify the installations:
node -v npm -v
You should see the installed versions of Node.js and npm displayed.
Alternatively, you can use yarn
, a popular alternative to npm. To install yarn
, run:
npm install -g yarn
Verify the installation with:
yarn -v
Setting Up a New Svelte Project Using the Svelte CLI or a Template
With Node.js and npm (or yarn) installed, you can now create a new Svelte project. There are two primary ways to set up a Svelte project: using the Svelte CLI or a template.
Using the Svelte CLI
- Install the Svelte CLI: Open your terminal and run:
npm install -g create-svelte
- Create a New Project: Navigate to the directory where you want to create your project and run it:
npx create-svelte my-svelte-app
Replacemy-svelte-app
with your desired project name. - Follow the Prompts: The CLI will prompt you to choose a project template and configure some options. Follow the instructions to complete the setup.
- Install Dependencies: Navigate to your project directory and install the necessary dependencies:
cd my-svelte-app npm install
If you preferyarn
, use:yarn install
Using a Template
- Clone a Template Repository: You can also start with a pre-configured template by cloning a repository. For example, to use the Svelte template, run:
npx degit sveltejs/template my-svelte-app
Replacemy-svelte-app
with your desired project name. - Install Dependencies: Navigate to your project directory and install the dependencies:
cd my-svelte-app npm install
Or withyarn
:yarn install
Configuring Your Code Editor (e.g., VS Code) for Optimal Svelte Development
A well-configured code editor can significantly enhance your productivity. Visual Studio Code (VS Code) is a popular choice among developers due to its extensive extension library and customization options. Here’s how to set up VS Code for Svelte development:
- Install VS Code: If you haven’t already, download and install Visual Studio Code.
- Install the Svelte Extension: Open VS Code, go to the Extensions view by clicking on the Extensions icon in the Activity Bar on the side of the window or by pressing
Ctrl+Shift+X
. Search for “Svelte” and install the official Svelte extension by Svelte. - Configure Settings: To ensure optimal performance and features, you may want to adjust some settings. Open the settings (
Ctrl+,
), and you can customize various options like auto-formatting, linting, and more. - Install Additional Extensions: Depending on your workflow, you might find these extensions helpful:
- Prettier: For code formatting.
- ESLint: For JavaScript/TypeScript linting.
- Live Server: For live-reloading during development.
By following these steps, you’ll have a robust development environment ready for building Svelte applications. With Node.js, npm (or yarn), a new Svelte project, and a well-configured code editor, you’re all set to start your journey with Svelte.
Understanding Svelte Fundamentals
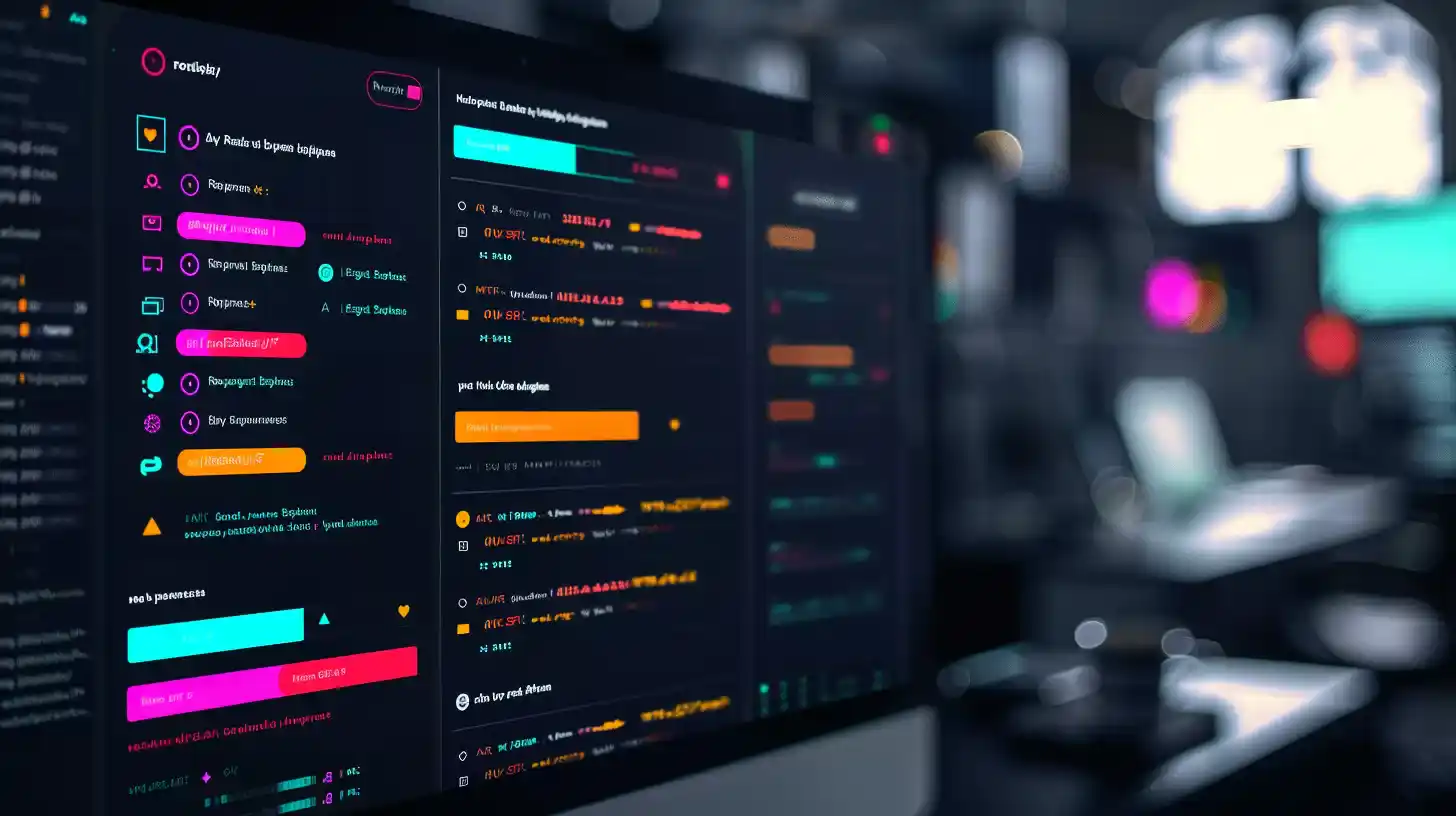
In this section, we’ll delve into the core concepts of Svelte, which will provide you with a solid foundation to build dynamic and efficient web applications. We’ll cover components, reactivity, DOM manipulation, conditional rendering and loops, and styling components.
Components
Components are the building blocks of a Svelte application. They encapsulate markup, styles, and behavior, making your code modular and reusable.
Defining and Structuring Svelte Components
A Svelte component is typically defined in a .svelte
file. Each component file contains three main sections: <script>
, <style>
, and the HTML markup.
<script>
let name = 'world';
</script>
<style>
h1 {
color: blue;
}
</style>
<h1>Hello {name}!</h1>
Importing and Using Components in Your Application
To use a component within another component, you need to import it and then include it in the markup.
<script>
import ChildComponent from './ChildComponent.svelte';
</script>
<ChildComponent />
Passing Data to Components Using Props
Props allow you to pass data from a parent component to a child component.
ParentComponent.svelte
<script>
import ChildComponent from './ChildComponent.svelte';
let message = "Hello from parent!";
</script>
<ChildComponent message={message} />
ChildComponent.svelte
<script>
export let message;
</script>
<p>{message}</p>
Reactivity
How Svelte’s Reactive System Works
Svelte’s reactivity system is one of its most compelling features, setting it apart from other frameworks and libraries. Unlike traditional approaches that rely on a virtual DOM, Svelte compiles your code into highly efficient, imperative JavaScript that updates the DOM when the state of your application changes. This means that Svelte applications can be faster and more efficient, with fewer abstractions and less boilerplate code.
At the core of Svelte’s reactivity is the idea that changes in state should automatically propagate through your application, updating the user interface as needed. This is achieved through a combination of reactive assignments, reactive statements, and derived values, which we’ll explore in more detail below.
Declaring Reactive Variables and Updating Them
In Svelte, any variable declared with the let
the keyword is inherently reactive. This means that when you update the value of a reactive variable, Svelte will automatically update the DOM to reflect the new state. Here’s a simple example to illustrate this concept:
<script>
let count = 0;
function increment() {
count += 1;
}
</script>
<button on:click={increment}>
Clicked {count} {count === 1 ? 'time' : 'times'}
</button>
In this example, the count
the variable is reactive. Each time the button is clicked, the increment
function updates count
, and Svelte automatically updates the button text to show the new count.
Reactive Statements and Derived Values
Svelte also provides a powerful feature called reactive statements, which allows you to declare that a block of code should be re-run whenever its dependencies change. Reactive statements are denoted by a dollar sign ($
) followed by a colon (:
) and are typically used to derive values from reactive variables.
Here’s an example:
<script>
let width = 100;
let height = 50;
$: area = width * height;
</script>
<p>The area is {area} square units.</p>
<input type="number" bind:value={width}>
<input type="number" bind:value={height}>
In this example, the area
variable is a derived value that automatically updates whenever width
or height
changes. The reactive statement $: area = width * height;
ensures that the calculation is re-run whenever its dependencies (width
and height
) are updated.
Reactive statements can also be used to perform side effects, such as logging values or making network requests:
<script>
let name = 'world';
$: console.log(`Hello, ${name}!`);
</script>
<input type="text" bind:value={name}>
In this case, the console.log
the statement will run every time the name
variable changes.
By leveraging Svelte’s reactivity system, you can build dynamic, responsive applications with minimal effort. The combination of reactive variables, reactive statements, and derived values allows you to express complex state transformations clearly and concisely, making your code easier to read, write, and maintain.
DOM Manipulation
When working with Svelte, understanding how to access and manipulate the DOM is crucial. This section will guide you through the essential techniques and concepts for effective DOM manipulation in Svelte applications.
Accessing and Manipulating the DOM with Svelte
Svelte provides a straightforward way to interact with the DOM directly within your components. Unlike other frameworks that might abstract away DOM manipulation, Svelte allows you to use native JavaScript methods to access and modify DOM elements.
Here’s a simple example of how you can access a DOM element using Svelte:
<script>
let myElement;
function changeText() {
myElement.textContent = 'Text has been changed!';
}
</script>
<div bind:this={myElement}>
Initial text
</div>
<button on:click={changeText}>Change Text</button>
In the example above, the bind:this
directive is used to bind the myElement
variable to the DOM element. This allows you to directly manipulate the element within your component’s script.
Using Lifecycle Functions
Svelte offers several lifecycle functions that allow you to run code at specific points in a component’s lifecycle. These functions are particularly useful for DOM manipulation, as they enable you to perform actions when a component is mounted, updated, or destroyed.
onMount
The onMount
function is called when a component is first rendered to the DOM. This is the perfect place to perform any setup that requires access to the DOM.
<script>
import { onMount } from 'svelte';
onMount(() => {
console.log('Component has been mounted');
// Perform DOM manipulations here
});
</script>
onDestroy
The onDestroy
function is called just before a component is removed from the DOM. This is useful for cleaning up resources such as event listeners or timers.
<script>
import { onDestroy } from 'svelte';
onDestroy(() => {
console.log('Component is about to be destroyed');
// Clean up DOM manipulations here
});
</script>
Handling Events and Event Modifiers
Handling events in Svelte is simple and intuitive. You can listen for events using the on:
directive and define event handlers directly within your component.
<script>
function handleClick() {
alert('Button clicked!');
}
</script>
<button on:click={handleClick}>Click Me</button>
Svelte also provides event modifiers that can be used to modify the behavior of event listeners. Some common event modifiers include:
preventDefault
: Prevents the default action associated with the event.stopPropagation
: Stops the event from propagating to parent elements.self
: Ensures the event handler is only called if the event originated from the target element itself.
<script>
function handleSubmit(event) {
event.preventDefault();
alert('Form submitted!');
}
</script>
<form on:submit|preventDefault={handleSubmit}>
<button type="submit">Submit</button>
</form>
In the example above, the preventDefault
modifier is used to prevent the form’s default submission behavior, allowing you to handle the submission entirely within your event handler.
By understanding and utilizing these DOM manipulation techniques, lifecycle functions, and event-handling strategies, you can create dynamic and interactive Svelte applications with ease.
Conditional Rendering and Loops
In any dynamic web application, the ability to conditionally render content and iterate over lists is crucial. Svelte provides intuitive and efficient ways to handle these needs with if/else
blocks and {#each}
blocks. Let’s explore how you can leverage these features to create dynamic and responsive user interfaces.
Conditionally Rendering Content with if/else
Blocks
Svelte makes conditional rendering straightforward with its if/else
syntax. This allows you to display content based on certain conditions, ensuring that your application reacts seamlessly to changes in state or user input.
Basic if
Block
The simplest form of conditional rendering is the if
block. Here’s an example of how you can use it:
<script>
let isLoggedIn = false;
</script>
{#if isLoggedIn}
<p>Welcome back, user!</p>
{/if}
In this example, the paragraph element will only be rendered if isLoggedIn
is true
.
if/else
Block
Often, you may want to render alternative content when the condition is not met. This is where the else
block comes in handy:
<script>
let isLoggedIn = false;
</script>
{#if isLoggedIn}
<p>Welcome back, user!</p>
{:else}
<p>Please log in to continue.</p>
{/if}
Here, if isLoggedIn
is false
, the message prompting the user to log in will be displayed instead.
elseif
Block
For more complex conditions, you can chain multiple conditions using elseif
:
<script>
let userRole = 'guest';
</script>
{#if userRole === 'admin'}
<p>Welcome, Admin!</p>
{:elseif userRole === 'editor'}
<p>Welcome, Editor!</p>
{:else}
<p>Welcome, Guest!</p>
{/if}
This example checks the value of userRole
and renders different content based on whether the user is an admin, editor, or guest.
Rendering Lists and Collections with {#each}
Blocks
When dealing with arrays or collections of data, Svelte’s {#each}
the block allows you to efficiently render lists. This feature is essential for displaying dynamically generated content, such as items fetched from an API or user-generated entries.
Basic {#each}
Block
Here’s a basic example of rendering a list of items:
<script>
let fruits = ['Apple', 'Banana', 'Cherry'];
</script>
<ul>
{#each fruits as fruit}
<li>{fruit}</li>
{/each}
</ul>
In this example, each item in the fruits
array is rendered as a list item (<li>
).
Accessing Index and Key
Sometimes, you may need to access the index of each item or provide a unique key for more efficient DOM updates. Svelte allows you to do this easily:
<script>
let fruits = ['Apple', 'Banana', 'Cherry'];
</script>
<ul>
{#each fruits as fruit, index (fruit)}
<li>{index + 1}: {fruit}</li>
{/each}
</ul>
Here, each fruit is displayed with its index, and the fruit itself is used as a unique key.
Nested {#each}
Blocks
Svelte also supports nested {#each}
blocks, which can be useful for rendering complex data structures:
<script>
let categories = [
{ name: 'Fruits', items: ['Apple', 'Banana', 'Cherry'] },
{ name: 'Vegetables', items: ['Carrot', 'Lettuce', 'Peas'] }
];
</script>
{#each categories as category}
<h2>{category.name}</h2>
<ul>
{#each category.items as item}
<li>{item}</li>
{/each}
</ul>
{/each}
In this example, each category is rendered with its list of items, demonstrating the flexibility and power of Svelte’s {#each}
block.
By mastering conditional rendering and loops, you can create dynamic, efficient, and user-friendly applications with Svelte. These tools are fundamental building blocks that will enable you to handle a wide range of scenarios in your web development projects.
Styling Components
Styling is an integral part of web development, and Svelte makes it straightforward to add styles to your components. This section will guide you through writing styles directly in Svelte components, using CSS preprocessors, and scoping styles to individual components.
Writing Styles Directly in Svelte Components
One of the most convenient features of Svelte is the ability to write styles directly within your component files. This approach keeps your styles encapsulated and closely tied to the components they affect.
To add styles to a Svelte component, you simply include a <style>
tag within your .svelte
file. Here’s an example:
<script>
let name = 'world';
</script>
<style>
h1 {
color: purple;
}
</style>
<h1>Hello {name}!</h1>
In this example, the h1
element is styled with a purple color. The styles defined in the <style>
tag only applies to the current component, ensuring that your styles do not accidentally affect other parts of your application.
Using CSS Preprocessors (e.g., SCSS, LESS)
While basic CSS is powerful, you might want to use CSS preprocessors like SCSS or LESS for more advanced styling capabilities, such as variables, nesting, and mixins. Svelte js supports these preprocessors out of the box with a bit of configuration.
To use SCSS in your Svelte project, follow these steps:
- Install the necessary packages:
npm install --save-dev svelte-preprocess sass
- Update your
svelte.config.js
:import sveltePreprocess from 'svelte-preprocess'; export default { preprocess: sveltePreprocess({ scss: { // Add any SCSS-specific configuration here }, }), };
- Write SCSS in your component:
<script> let name = 'world'; </script> <style lang="scss"> $primary-color: purple; h1 { color: $primary-color; } </style> <h1>Hello {name}!</h1>
With this setup, you can now use SCSS features within your Svelte native components. The process for using LESS is similar; just replace sass
with less
in the installation command and configuration.
Scoping Styles to Individual Components
Svelte automatically scopes styles to the component in which they are defined. This means that styles written in a component’s <style>
tag will not leak out and affect other components, providing a high level of encapsulation.
Svelte achieves this by adding unique class names to elements and their corresponding styles. For example:
<style>
h1 {
color: purple;
}
</style>
<h1>Hello world!</h1>
In the compiled output, Svelte will transform this to include a unique class:
<style>
h1.svelte-abc123 {
color: purple;
}
</style>
<h1 class="svelte-abc123">Hello world!</h1>
This automatic scoping ensures that your component styles remain modular and do not interfere with other parts of your application. If you need to apply global styles, you can use the :global
modifier:
<style>
:global(body) {
margin: 0;
font-family: Arial, sans-serif;
}
</style>
In this example, the styles within the :global
block are applied globally, affecting the entire document.
By understanding and utilizing these styling techniques, you can create well-organized, maintainable, and visually appealing Svelte applications.
Building a Simple Svelte Application
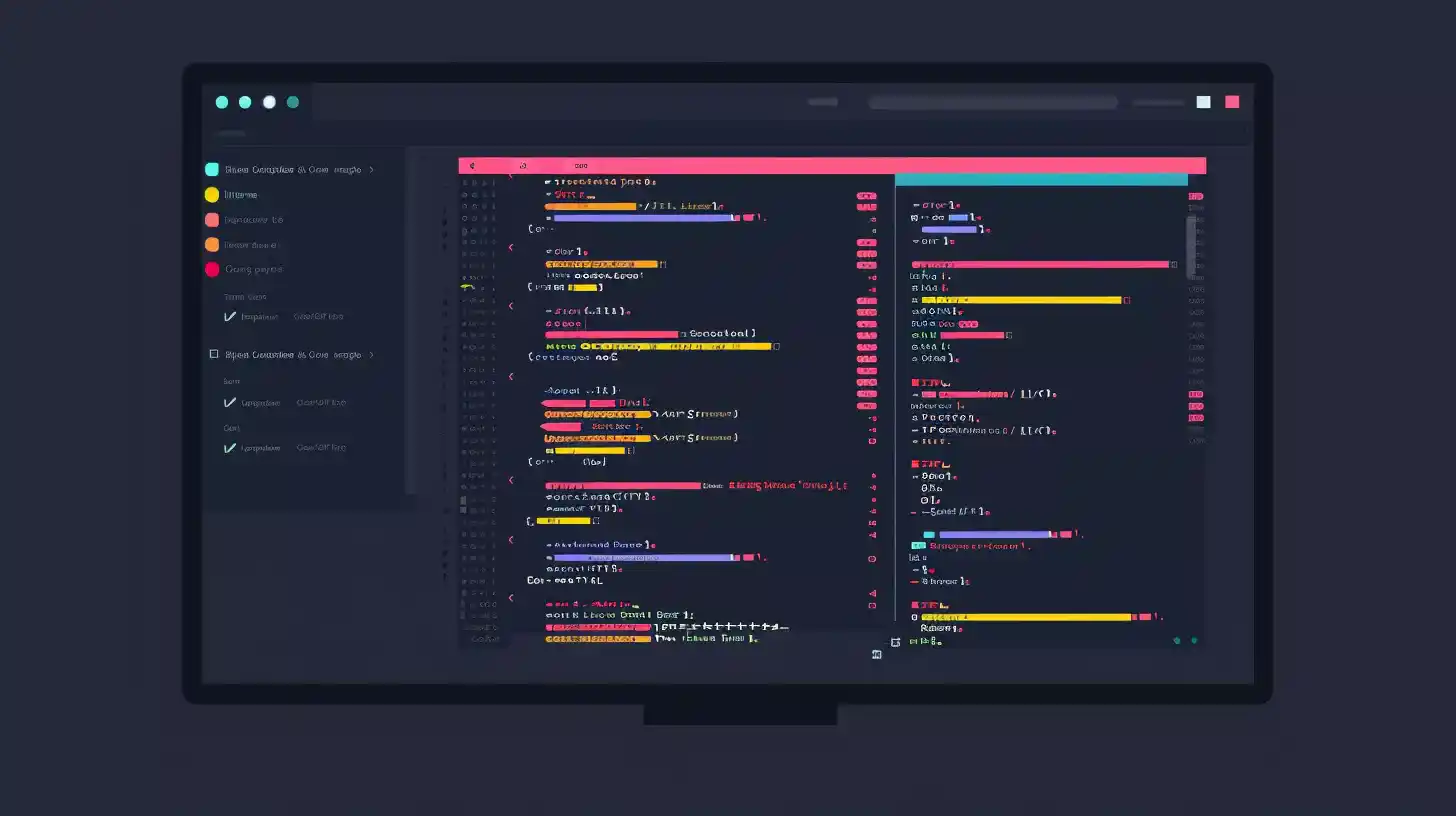
Embarking on creating a Svelte application is an exciting journey that combines simplicity and powerful features. This section will guide you through setting up your project, developing key components, and deploying your application for the world to see.
Project Setup
To start building your Svelte application, you’ll first need to set up a new project. Follow these steps:
- Creating a New Svelte Project:
- Open your terminal and run the following command to create a new Svelte project using the Svelte template:
npx degit sveltejs/template my-svelte-app cd my-svelte-app npm install
- This command clones the official Svelte template and installs the necessary dependencies.
- Open your terminal and run the following command to create a new Svelte project using the Svelte template:
- Organizing Your Project Structure:
- The default structure of a Svelte project is straightforward:
my-svelte-app/ ├── public/ ├── src/ │ ├── App.svelte │ ├── main.js ├── package.json ├── rollup.config.js └── ...
- The
src
directory contains your application’s source code, withApp.svelte
being the main component. - The
public
directory holds static assets like images and theindex.html
file.
- The default structure of a Svelte project is straightforward:
Developing the Application
With your project set up, it’s time to dive into development:
- Building the Main Application Component:
- Open
src/App.svelte
and start by defining your main component structure. For example:<script> let name = 'world'; </script> <style> h1 { color: #ff3e00; } </style> <h1>Hello {name}!</h1>
- This basic example demonstrates Svelte’s simplicity with embedded HTML, CSS, and JavaScript.
- Open
- Implementing Features like State Management and User Interactions:
- Svelte makes state management intuitive. For instance, you can create a simple counter:
<script> let count = 0; function increment() { count += 1; } </script> <button on:click={increment}> Count: {count} </button>
- This code snippet shows how to create a reactive counter and handle user interactions with event binding.
- Svelte makes state management intuitive. For instance, you can create a simple counter:
- Integrating Third-Party Libraries (if needed):
- To enhance your application, you might want to integrate third-party libraries. For example, adding a date-picker:
npm install svelte-datepicker
- Then, use it in your component:
<script> import DatePicker from 'svelte-datepicker'; let selectedDate; </script> <DatePicker bind:date={selectedDate} />
- To enhance your application, you might want to integrate third-party libraries. For example, adding a date-picker:
Deploying Your Application
Once your application is ready, it’s time to deploy it so others can access it:
- Building and Bundling Your Svelte Application:
- Run the following command to create a production build:
npm run build
- This command generates optimized files in the
public/build
directory, ready for deployment.
- Run the following command to create a production build:
- Hosting Options:
- There are several hosting options available:
- Netlify:
- Connect your repository to Netlify, and it will automatically build and deploy your application.
- Vercel:
- Similar to Netlify, Vercel offers seamless integration with GitHub and automatic deployments.
- GitHub Pages:
- You can deploy your Svelte app to GitHub Pages by pushing your build files to a
gh-pages
branch.
- You can deploy your Svelte app to GitHub Pages by pushing your build files to a
- Netlify:
- There are several hosting options available:
To deploy on GitHub Pages, you can use the gh-pages
package:
npm install gh-pages --save-dev
Add the following scripts to your package.json
:
"scripts": {
"predeploy": "npm run build",
"deploy": "gh-pages -d public"
}
Then, run:
npm run deploy
By following these steps, you’ll have your Svelte application up and running, and accessible to users worldwide.
With this comprehensive guide, you’re well-equipped to build, develop, and deploy a simple Svelte application. Continue exploring and expanding your skills to create more complex and powerful applications.
Project Setup
Creating a New Svelte Project
Getting started with Svelte is remarkably straightforward, thanks to its user-friendly setup process. To create a new Svelte project, follow these steps:
- Install Node.js and npm: Ensure you have Node.js and npm (Node Package Manager) installed on your machine. You can download and install them from nodejs.org.
- Create a New Project: Open your terminal or command prompt and run the following command to create a new Svelte project using the Svelte CLI:
npx degit sveltejs/template my-svelte-app cd my-svelte-app npm install
This command does the following:npx degit sveltejs/template my-svelte-app
: Clones the official Svelte template into a new directory namedmy-svelte-app
.cd my-svelte-app
: Navigate into the newly created project directory.npm install
: Installs the necessary dependencies for your Svelte project.
- Start the Development Server: Once the installation is complete, start the development server by running:
npm run dev
This command will start the Svelte development server and open your new project in your default web browser. You should see a welcome message from Svelte, indicating that your project is set up correctly.
Organizing Your Project Structure
A well-organized project structure is key to maintaining and scaling your application efficiently. Here’s a typical structure for a Svelte project:
my-svelte-app/
├── public/
│ ├── global.css
│ ├── favicon.png
│ └── index.html
├── src/
│ ├── assets/
│ │ └── (images, fonts, etc.)
│ ├── components/
│ │ └── (reusable Svelte components)
│ ├── routes/
│ │ └── (route-specific components)
│ ├── App.svelte
│ ├── main.js
│ └── (other directories and files as needed)
├── .gitignore
├── package.json
├── rollup.config.js
└── README.md
- public/: Contains static files such as global stylesheets, images, and the main
index.html
file. - src/: The main source directory for your application.
- assets/: A place to store static assets like images and fonts.
- components/: A directory for reusable Svelte components.
- routes/: If your application uses routing, this directory can store route-specific components.
- App.svelte: The root Svelte component of your application.
- main.js: The entry point for your application where the root component is initialized.
- .gitignore: Specifies files and directories that should be ignored by Git.
- package.json: Contains metadata about your project and its dependencies.
- rollup.config.js: Configuration file for Rollup, the module bundler used by Svelte.
- README.md: A markdown file that provides an overview of your project.
By following this structure, you ensure that your project remains clean and manageable as it grows. Feel free to adjust the structure to suit the specific needs of your application.
Developing the Application
Building the Main Application Component
To start developing your Svelte application, you’ll first need to create the main application component. This component will serve as the entry point for your app and will often be named App.svelte
. Here’s a simple example:
<script>
let message = "Welcome to Svelte!";
</script>
<main>
<h1>{message}</h1>
</main>
<style>
main {
text-align: center;
padding: 1em;
max-width: 240px;
margin: 0 auto;
}
h1 {
color: #ff3e00;
}
</style>
In this example, we define a message
variable in the <script>
tag and use it within the HTML structure of our component. The styles are scoped to this component, ensuring they don’t affect other parts of the application.
Implementing Features like State Management and User Interactions
Svelte makes state management and handling user interactions straightforward. You can declare reactive variables and create functions to update them. Here’s how you can manage state and handle a button click event:
<script>
let count = 0;
function increment() {
count += 1;
}
</script>
<main>
<h1>Count: {count}</h1>
<button on:click={increment}>Increment</button>
</main>
<style>
main {
text-align: center;
padding: 1em;
max-width: 240px;
margin: 0 auto;
}
button {
padding: 0.5em 1em;
font-size: 1em;
color: white;
background-color: #ff3e00;
border: none;
border-radius: 5px;
cursor: pointer;
}
button:hover {
background-color: #ff5722;
}
</style>
In this example, we declare a count
variable and an increment
function to update the count. The on:click
directive binds the button click event to the increment
function, allowing the count to increase each time the button is clicked.
Integrating Third-Party Libraries (if needed)
At times, you might need to integrate third-party libraries to extend the functionality of your Svelte application. For example, you might want to use a date picker library. Here’s how you can integrate a third-party library:
- Install the library: Use npm or yarn to add the library to your project. For example, to install
flatpickr
:npm install flatpickr
- Import and use the library: Import the library in your Svelte component and initialize it. Here’s an example of using
flatpickr
:<script> import flatpickr from "flatpickr"; import "flatpickr/dist/flatpickr.css"; let datepicker; onMount(() => { flatpickr(datepicker, { enableTime: true, dateFormat: "Y-m-d H:i", }); }); </script> <input type="text" bind:this={datepicker} /> <style> /* Add any additional styles for the date picker here */ </style>
In this example, we import flatpickr
and its CSS, then use the onMount
lifecycle function to initialize the date picker on the input element.
By following these steps, you can build the main components of your Svelte application, manage state, handle user interactions, and integrate third-party libraries to enhance functionality. This foundation will enable you to develop a robust and interactive Svelte application.
Deploying Your Application
After you’ve built your Svelte application and ensured it runs smoothly in your development environment, the next step is to deploy it so that users can access it online. This section will guide you through the process of building and bundling your Svelte application, as well as exploring various hosting options.
Building and Bundling Your Svelte Application
Before deploying your application, you need to build and bundle it for production. This process optimizes your code, making it more efficient and faster to load. Here’s how you can do it:
- Run the Build Command: Navigate to your project directory in the terminal and run the following command:
npm run build
This command will generate apublic
directory containing the optimized and minified versions of your application files. - Inspect the Output: After the build process is complete, inspect the contents of the
public
directory. You should see your HTML, CSS, and JavaScript files, along with any assets your application uses. - Test Locally: Before deploying, it’s a good practice to test the production build locally. You can use a simple static server to serve the contents of the
public
directory. For example, you can use theserve
package:npx serve public
Open your browser and navigate to the provided URL to ensure everything works as expected.
Hosting Options
With your application built and ready, the next step is to choose a hosting provider. There are several options available, each with its own set of features and advantages. Here are three popular choices:
- Netlify: Netlify is a powerful and user-friendly platform for deploying static sites. It offers continuous deployment, custom domains, and serverless functions.
- Steps to Deploy:
- Sign up for a Netlify account.
- Connect your Git repository to Netlify.
- Configure the build settings (usually, the default settings work fine for Svelte projects).
- Deploy your site and get a live URL.
- Steps to Deploy:
- Vercel: Vercel is another excellent option for hosting static sites and serverless functions. It is known for its simplicity and seamless integration with front-end frameworks.
- Steps to Deploy:
- Sign up for a Vercel account.
- Import your project from a Git repository.
- Vercel will automatically detect your Svelte project and configure the build settings.
- Deploy your site and obtain a live URL.
- Steps to Deploy:
- GitHub Pages: GitHub Pages is a free hosting service provided by GitHub for hosting static sites directly from a GitHub repository.
- Steps to Deploy:
- Push your built application to a GitHub repository.
- Go to the repository settings on GitHub.
- Under the “Pages” section, select the branch and folder (e.g.,
main
branch and/public
folder) to deploy. - Save the settings and GitHub Pages will generate a live URL for your site.
- Steps to Deploy:
Each of these hosting options has its unique features and benefits. Choose the one that best fits your needs and follow the steps to get your Svelte application online.
By following these instructions, you can seamlessly transition from development to deployment, ensuring that your Svelte application is accessible to users around the world. Happy deploying!
Resources and Next Steps
As you continue your journey into Svelte development, there are numerous resources and advanced topics you can explore to deepen your understanding and enhance your skills. This section will guide you through some recommended learning materials, advanced concepts, and tips for continuous improvement.
Recommended Svelte Learning Resources
To build a solid foundation and stay updated with the latest in Svelte, consider the following resources:
- Official Svelte Documentation: The Svelte docs are the most authoritative source of information. It provides comprehensive Svelte-docs, API references, and examples to help you understand every aspect of Svelte.
- Tutorials: The Svelte tutorial on the official website offers a hands-on approach to learning. It covers the basics and gradually introduces more complex topics.
- Books: “Svelte and Sapper in Action” by Mark Volkmann is an excellent book that covers both Svelte and its companion framework, Sapper. It’s a great resource for learning how to build full-fledged applications.
- Online Courses: Platforms like Udemy, Pluralsight, and Frontend Masters offer courses on Svelte. These courses range from beginner to advanced levels and provide in-depth knowledge through video tutorials.
- Community and Forums: Join the Svelte Discord community and forums like Stack Overflow to connect with other developers, ask questions, and share knowledge.
Exploring Advanced Svelte Topics
Once you’re comfortable with the basics, you can delve into advanced topics to harness the full power of Svelte:
- Stores: Learn about Svelte stores, which are a simple and reactive way to manage state across your application. The official documentation on Svelte stores is a good starting point.
- Transitions and Animations: Svelte’s built-in transition and animation features allow you to create smooth and visually appealing effects with minimal effort. Explore the transitions and animations documentation to get started.
- Custom Directives and Actions: Understand how to create custom directives and actions for more complex DOM manipulation and behavior. The actions documentation provides detailed guidance.
- SSR and Sapper: If you’re interested in server-side rendering (SSR) and building more complex applications, explore Sapper, the Svelte framework for SSR. The Sapper documentation will guide you through the process.
Tips for Further Improving Your Svelte Development Skills
Continuous improvement is key to becoming proficient in Svelte. Here are some tips to help you on your journey:
- Build Real Projects: The best way to learn is by doing. Start with small projects and gradually take on more complex ones. This hands-on experience will solidify your understanding and expose you to real-world challenges.
- Contribute to Open Source: Contributing to open-source Svelte projects on platforms like GitHub can provide valuable experience and help you connect with the community.
- Stay Updated: Follow the Svelte blog and subscribe to newsletters like “Svelte Society” to stay informed about the latest updates, features, and best practices.
- Practice Code Reviews: Reviewing code written by others and having your code reviewed can provide new perspectives and insights. Participate in code review sessions within the Svelte community.
- Experiment and Innovate: Don’t be afraid to experiment with new ideas and approaches. Innovation often comes from trying out unconventional methods and learning from the outcomes.
By leveraging these resources and continually challenging yourself, you’ll be well on your way to mastering Svelte and building powerful, efficient web applications.
Conclusion
In this guide, we’ve embarked on a journey to understand and utilize Svelte for web development. We’ve explored the foundations of what makes Svelte a compelling choice, from its simplicity and performance to its exceptional developer experience. Here’s a recap of the key points we’ve covered:
- Introduction to Svelte: We began by discussing what Svelte is and why it stands out in the crowded landscape of web development frameworks.
- Setting Up Your Development Environment: We walked through the steps to install Node.js and npm, set up a new Svelte project, and configure your code editor for an optimal development experience.
- Understanding Svelte Fundamentals: We delved into the core concepts of Svelte, including components, reactivity, DOM manipulation, conditional rendering, loops, and styling.
- Building a Simple Svelte Application: We guided you through the process of creating and organizing a new Svelte project, developing the main application component, and implementing essential features.
- Deploying Your Application: We discussed how to build and bundle your Svelte application and explored various hosting options to get your project online.
- Resources and Next Steps: We provided recommendations for further learning, including documentation, tutorials, and books, and highlighted advanced topics to explore as you continue your Svelte journey.
As you move forward, we encourage you to start building your own Svelte projects. Experiment with the concepts you’ve learned, and don’t hesitate to dive deeper into advanced topics. The Svelte community is vibrant and supportive, offering a wealth of resources to help you grow as a developer. Keep learning, keep building, and enjoy the simplicity and power that Svelte brings to your web development endeavors.